I’m a big fan of helper classes. They help make your code reusable and easier to read. Here are some of my favourite C# helper classes.
Camera
What is it?
A method to allow you take screenshots on demand that gives the files meaningful names.
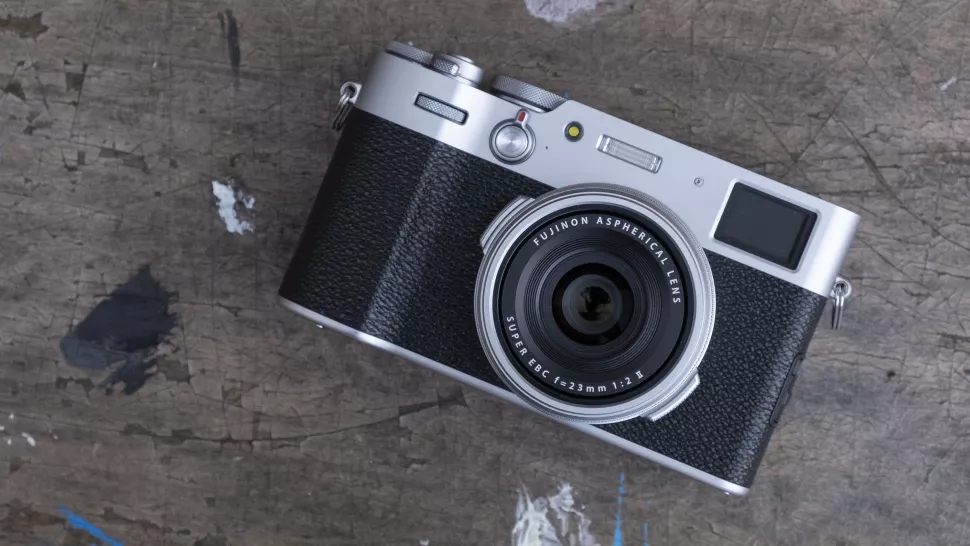
Why do I need it?
Taking screenshots at point of failure is extremely valuable when trying to understand why a test failed. Adding this method allows you to add that to your teardown process but also to go a step further and take screenshots at specific points during a test to aid in debugging issues.
What does the code look like?
public static void TakeScreenshot()
{
var screenshotImage = ((ITakesScreenshot)Browser.Driver).GetScreenshot();
var title = ScenarioContext.Current.ScenarioInfo.Title;
var runName = title + " " + DateTime.Now.ToString("yyyy-MM-dd-HH_mm_ss");
screenshotImage.SaveAsFile(runName + ".jpg", ScreenshotImageFormat.Jpeg);
}
Download
What is it?
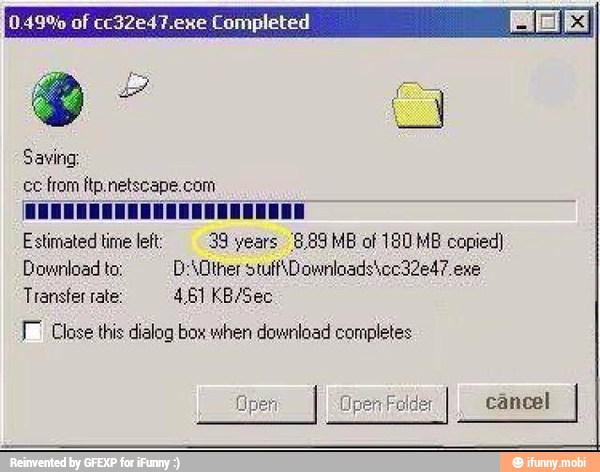
The downloads helper creates an array with the file names of downloaded files of a particular type and provides a method to delete the files at the end of the test.
Why do I need it?
For tests that download files, this helper provides you with the tools needed to validate the files downloaded correctly and to remove them at the end of the test. When implemented correctly, it will even delete files when the test fails.
What does the code look like?
public static string[] GetFileFromDownloadFolder(string fileType)
{
var fList = new List<string>();
var files = Directory.GetFiles(Browser.DownloadFolder, $"*.{fileType}");
foreach (var file in files) fList.Add(Path.GetFileName(file));
return fList.ToArray();
}
public static void DeleteFiles(string fileType)
{
var files = Directory.GetFiles(Browser.DownloadFolder, $"*.{fileType}");
foreach (var file in files) File.Delete(file);
}
File Path
What is it?
This helper method gives you a reusable root directory for interacting with files for upload and download.
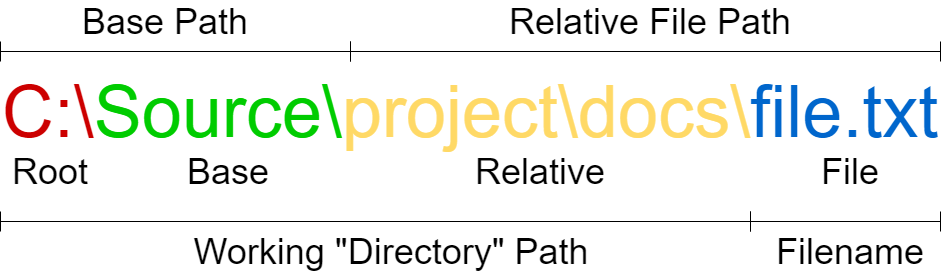
Why do I need it?
If you need to test file uploads or downloads, the FilePath helper removes the need for hardcoded paths and makes tests compatible with running on build agents etc.
What does the code look like?
public static string BasePlus(string additionalPath)
{
var basePath = AppDomain.CurrentDomain.BaseDirectory;
return Path.Combine(basePath, $"{additionalPath}");
}
Key Sender
What is it?
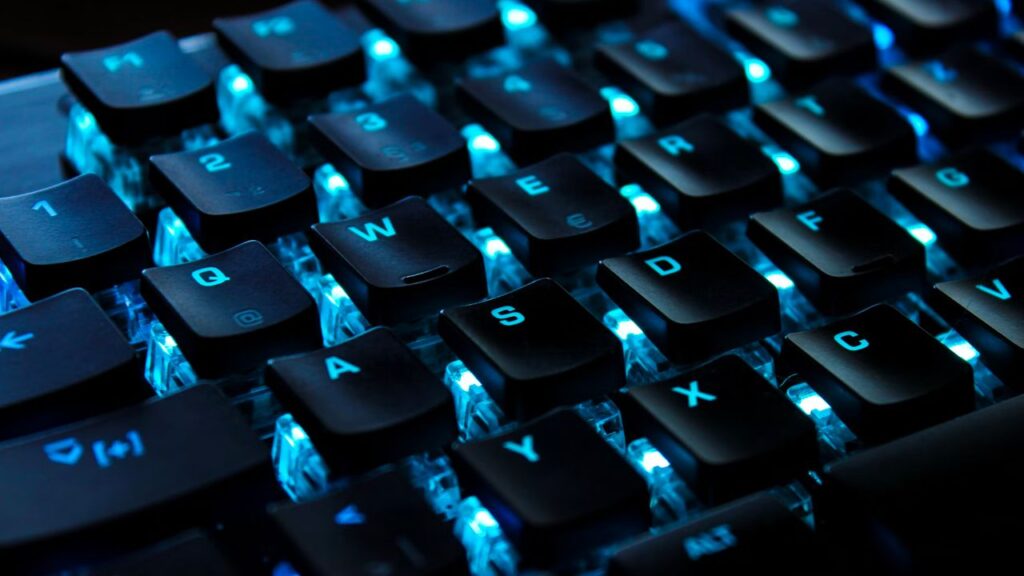
A reusable implementation of IWebDrivers SendKeys.
Why do I need it?
When you have tests that fill in forms you want them to do so in a reliable, consistent way and normally with random but meaningful data. Using a Key Sender helper method gives you that without having to add yous data generators to every class. For this class I recommend using ParkSquare Testing Helpers and Faker.Net for your random data generation – with these you can then generate as many different helpers as your needs require.
What does the code look like?
This will vary depending on your specific needs but a couple of examples are below
public void Lipsum(IWebElement element)
{
element.SendKeys(LipsumGenerator.Generate(1));
}
public void Date(IWebElement element)
{
element.SendKeys(DateTime.Now.ToString("dd/MM/yyyy"));
}
public void Forename(IWebElement element)
{
element.SendKeys(NameGenerator.AnyForename());
}
Wait
What is it?
The wait class is probably one of my most used. It provides me with all the different ways to wait for something to happen that I could possibly need.
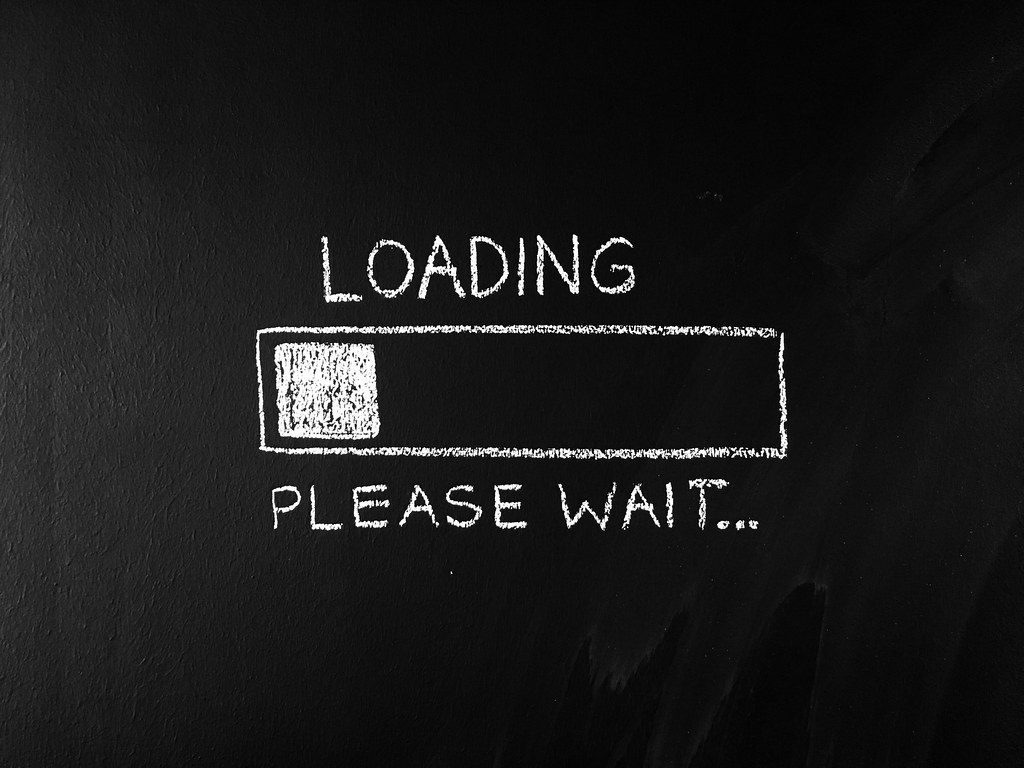
Why do I need it?
At some point, everyone runs into a point with a test where we need to wait for some action to happen, whether its for an element to load, an action to complete, or just to add some support for a system that runs slow occasionally. The variety of options are endless, but below are a couple of examples of Wait helpers you might want to use.
What does the code look like?
Want a test to wait until an element is available to be clicked? This helper does that and even provides an option for an extended wait time.
private static void WaitForElementToBeClickable(IWebElement element, bool defaultTimeout = true)
{
const int defaultWaitTime =30;
const int extendedWaitTime = 60;
var wait = new WebDriverWait(Browser.Driver,
TimeSpan.FromSeconds(defaultTimeout ? defaultWaitForObject : extendedWaitTime));
wait.Until(ExpectedConditions.ElementToBeClickable(element));
}
You can have much simpler implementations where you just wait for a fixed time period instead, this is less advisable, but can be useful when debugging. In this example we force the addition of a string to explain why it is being used.
public static void ForSeconds(int seconds, string reason)
{
Thread.Sleep(TimeSpan.FromSeconds(seconds));
}
Browser Tabs
What is it?
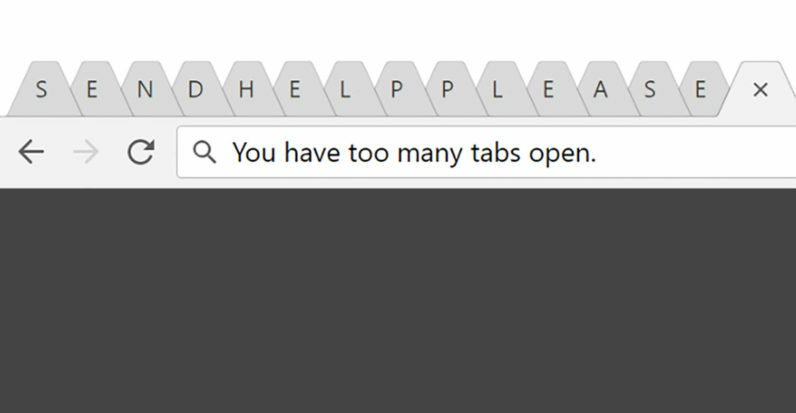
Some might consider this excessive as much of these functions exist within IWebDriver already. I find by adding functions, like switching between windows and switching between iframes, to the helper they are much nicer to read in-line. It also helps keeps our code consistent for when we call more specialised helpers like the one that waits for a new window to open.
Why do I need it?
Do your tests ever open a link that opens a new tab or window? Then this helper class helps make your code neater and easier to read.
What does the code look like?
public static void SwitchToFirst()
{
Browser.Driver.SwitchTo().Window(Browser.Driver.WindowHandles.First());
}
public static void SwitchToLast()
{
Browser.Driver.SwitchTo().Window(Browser.Driver.WindowHandles.Last());
}
public static void SwitchToFrame(string frameName)
{
Browser.Driver.SwitchTo().Frame(frameName);
}
public static void WaitUntilNewWindowIsOpened(this IWebDriver driver, int expectedNumberOfWindows,
int maxRetryCount = 1200)
{
int returnValue;
bool boolReturnValue;
for (var i = 0; i < maxRetryCount; Wait.ForMilliseconds(100), i++)
{
returnValue = driver.WindowHandles.Count;
boolReturnValue = returnValue == expectedNumberOfWindows;
if (boolReturnValue) return;
}
//try one last time to check for window
returnValue = driver.WindowHandles.Count;
boolReturnValue = returnValue == expectedNumberOfWindows;
if (!boolReturnValue) throw new ApplicationException("New window did not open.");
}
Want more?
If you enjoyed this, be sure to check out my post The Shadow DOM isn’t a Marvel thing? about writing an extension method for IWebDriver.
Subscribe to The Quality Duck
Did you know you can now subscribe to The Quality Duck? Never miss a post but getting them delivered direct to your mailbox whenever I create a new post. Don’t worry, you won’t get flooded with emails, I post at most once a week.
0 Comments
1 Pingback